Types of Inheritance in Java
In the previous blog we learned about Inheritance in Java. If you want to visit the blog visit Inheritance in Java In this blog, we will go through the different types of inheritance supported in Java such as:
- Single Inheritance
- Multilevel inheritance
- Hierarchical Inheritance
- Multiple Inheritance(using Interface)
- Hybrid Inheritance(using Inheritance)
Note that in Java multiple inheritance and hybrid inheritance are not supported in Java through class. They are supported through interface. In order to show the inheritance extends keyword is used. When the extend keyword is used, it means that the child class is inheriting the properties of the parent class. For example if there are two classes class X and class Y and extends keyword is used, then it means that class Y is inheriting the properties of class X. Here class X is a parent class or superclass and class Y is the child class or subclass. We will go through the types of Inheritance in detail
Single Inheritance
Single Inheritance is very easy to understand. When one class inherits(extends) another class then it is called single inheritance.
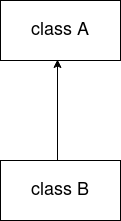
In the above figure class A is a parent class or superclass and class B is child class or subclass. class B is inheriting all the properties(methods,variables,..) of class A.
Single Inheritance Example:
AnimalExample.java:
DogExample.java
SingleInheritanceExample.java
Output:
Multilevel Inheritance
When a class inherits(extends) the other class, which again inherits(extends) another class is known as Multilevel Inheritance For example there are three classes X, Y and Z, class Z extends class Y and class Y extends class X.
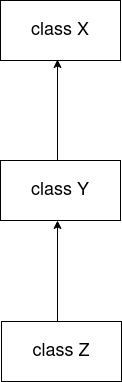
Multilevel Inheritance Example:
AnimalExample.java:
DogExample.java
BabyDogExample.java:
MultilevelInheritanceExample.java
Output:
Hierarchical Inheritance
Hierarchical Inheritance in Java means when one class is inherited(extended) by its child classes then it is called Hierarchical Inheritance. For example suppose there are four classes: class W, class X, class Y and class Z. class W is a parent calls of X, Y and Z.
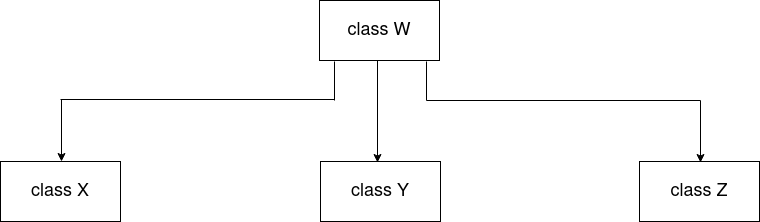
Hierarchical Inheritance Example:
AnimalExample.java:
DogExampe.java:
CatExampe.java:
HierarchicalInhertianceExample.java:
Output:
Why Java doesn't support multiple inheritance?
In order to reduce the complexity and increase the simplicity, Java doesn’t support multiple inheritance because it creates ambiguity. Suppose if we consider three classes X, Y and Z and class Z inherits class X and Y, and if class X and class Y have the same method defined in them and if we call it from the child class, then there will be ambiguity and the compiler will not understand which method to call, whether to call the method from class X or from class Y. So the compiler gives an error and the program gets terminated. For example:
X.java:
Y.java:
Z.java:
Multiple Inheritance
When one class inherits(extends) multiple classes is called multiple inheritance. But in Java multiple inheritance is not supported. But it is possible using the interface, we will see it in our upcoming blogs.
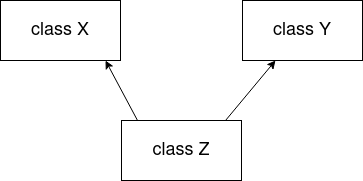
Hybrid Inheritance
Hybrid Inheritance in Java is nothing but the combination of single and multiple inheritance. But it is also supported through the interface.
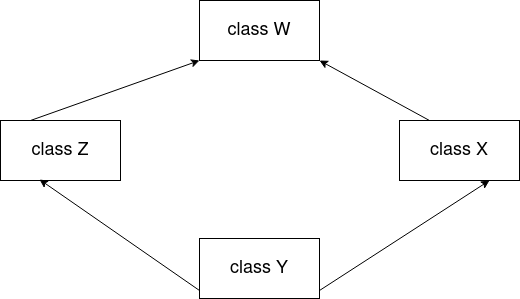
Access Modifiers in Java
Access Modifiers in Java define the scope of fields, methods, class or constructor. By applying the access modifier, we can change the access level of class, fields, methods of constructors. Following are access modifiers:
- private
- default
- protected
- public
- private
- default
- protected
- public
private access modifier in java means when we define a field or method as private then we will not be able to access that outside the class, we can only access them within that class. For better understanding we will go through the example:
In the above example there are two classes AtrowelPrivateModifierEx and TestPrivateModifierEx in which there is one private data field and one private method. You can see that we are trying to access the data field and method using the other class, which in return gives error, because as we have seen earlier that private fields and methods are accessible only within its class and we are trying to access it in another class.Now we will see another example where we have defined the constructor as private and will see how the code behave.
In the above example we have defined the constructor as private due to which we will not be able to create an instance of the class from outside of the class, because we have defined the constructor as private. Please make a note that the class cannot be private or protected except its child classes.
default access modifier in java is when we don’t use any modifier, then it is treated as default by default. When we use default for fields, methods or class then they are accessible only within the same package. They cannot be accessible outside the package. It gives more accessibility than private modifier, but it is more restrictive as compared to public and protected. For example:
In the above example we can see that we have defined two classes in different packages and we are trying to access the class DefaultModifierEx outside of its package, as the class is not public so it will not be accessible and will give a compilation error.
protected access modifier in java is accessible within its package and outside of its package but only through inheritance. It can be applied onIt gives more accessibility than default. For example:
ProtectedModifierEx.java
ProtectedModifier.java
Output:
In the above example we have created two packages named atrowelpack and atrowelpackage. class ProtectedModifierEx class of package atrowelpack is public, so we can access it from outside the package. But the method displayMsg() from the same package is declared as protected, so we can access it from outside of the package only through the inheritance.
public access modifier in java is the modifier that is accessible everywhere. It can be accessible in the class, outside the class, within the package ,outside the package. For example:
PublicModifierEx.java
PublicModifier.java
Output:
Java Access Modifiers Table
The following table will give you the better understanding of the access modifiers
Access Modifiers | Accessed within the class | Accessed within the package | Accessed outside package by child class only | Accessed outside the package |
---|---|---|---|---|
private | Yes | No | No | No |
default | Yes | Yes | No | No |
protected | Yes | Yes | Yes | No |
public | Yes | Yes | Yes | Yes |