JavaScript Introduction
In this blog, we will look into the overview of JavaScript, where it came from, how it works, and what kinds of things you can accomplish with it.
What is JavaScript
JavaScript is the Web programming language. It is used by the vast majority of modern websites, and all modern web browsers—on desktops, game consoles, tablets, and smartphones—include JavaScript interpreters, making JavaScript the most widely used programming language in history.
JavaScript is one of three technologies that all Web developers must learn: HTML to specify web page content, CSS to specify web page presentation, and JavaScript to provide web page behavior. The term "JavaScript" is slightly misleading. Except for a superficial syntactic resemblance, JavaScript is fundamentally distinct from the Java programming language.
JavaScript has long beyond its origins as a scripting language to become a robust and efficient general-purpose language. The language's most recent version (see the sidebar) defines additional features for serious large-scale software development. JavaScript can be used for both client and server-side development.
JavaScript is a declarative and imperative language. JavaScript has a standard object library such as Array, Date, and Math, as well as a basic set of language components such as operators, control structures, and statements.
Client-Side JavaScript
Client-side JavaScript is a programming language that runs in the user's web browser. It is used to add interactivity and dynamic content to web pages. Client-side JavaScript can be used to do things like:
- Validate form input
- Create animations and effects
- Add interactive features to maps and charts
- Make AJAX requests to the server
- Implement drag-and-drop functionality
- Handle user events like clicks, scrolls, and mouse movements
Client-side JavaScript is a powerful tool that can be used to create engaging and interactive web experiences. It is a must-know skill for any web developer. Here are some examples of client-side JavaScript:
- A form validation script that checks if the user has entered a valid email address.
- An animation that changes the color of a button when the user hovers over it.
- A map that allows the user to zoom in and out and pan around.
- A chart that updates in real-time as the user enters data.
- An AJAX request that fetches data from the server and updates the web page without reloading it.
- A drag-and-drop feature that allows the user to rearrange elements on a web page.
- A user event handler that plays a sound when the user clicks on a button.
Server-Side JavaScript
Server-side JavaScript is a programming paradigm that uses JavaScript to execute code on the server instead of the client. This allows developers to create dynamic and interactive web applications that can respond to user input in real-time.
Server-side JavaScript is becoming increasingly popular due to its many advantages, including:
- Single-codebase development: Server-side JavaScript allows developers to write code once and run it on both the server and the client. This can save time and effort, and it also helps to ensure that the code is consistent across all platforms.
- Asynchronous programming: Server-side JavaScript is asynchronous, which means that it can handle multiple requests at the same time. This makes it ideal for applications that need to be able to handle a lot of traffic.
- Ease of use:JavaScript is a popular and well-known language, so many developers are already familiar with it. This makes it a good choice for server-side development, as it can help to reduce the learning curve for new developers.
Some of the popular frameworks for server-side JavaScript include:
- Node.js: Node.js is a popular JavaScript runtime environment that can be used to create both server-side and client-side applications. It is known for its asynchronous event-driven architecture, which makes it efficient for handling a lot of traffic.
- Express.js: Express.js is a popular web framework for Node.js. It provides a simple and elegant API for creating web applications.
- Meteor: Meteor is a full-stack JavaScript framework that can be used to create web, mobile, and desktop applications. It is known for its real-time capabilities and its ability to scale to large applications.
Server-side JavaScript is a powerful and versatile language that can be used to create a wide variety of web applications. If you are looking for a language that is easy to learn, efficient, and scalable, then server-side JavaScript is a great option.
How to Include a JavaScript File in HTML?
There are two ways wherein JavScript can be included
Inline JavaScript:
This is the simplest approach to link a JavaScript file. Simply place the JavaScript code within the <script> tag in our HTML file to accomplish this. The <script> tag has two attributes: src and type. The src attribute indicates the path to the JavaScript file, and the src attribute specifies the file type, which is always text/javascript.
Consider this Inline JavaScript example:
Output:
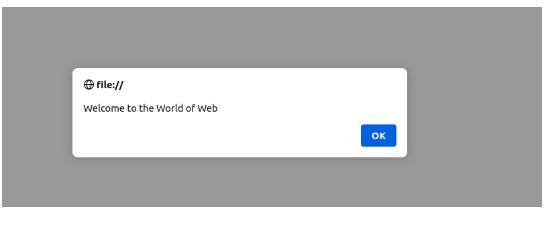
External JavaScript File
If the JavaScript code is in a separate.js file, then we must proceed as follows:
- Create a new js file and write the JavaScript code into it. Assume the JavaScript file is called scriptExample.js.
- In the HTML file, use the <script> element with the src attribute to link to the external JavaScript file. Place this element inside the <head> or at the end of the <body> section of the HTML, just before the closing </body> tag.
Let's examine an example of importing an external JavaScript file using the <head> tag:
index.js
scriptExample.js
Output:
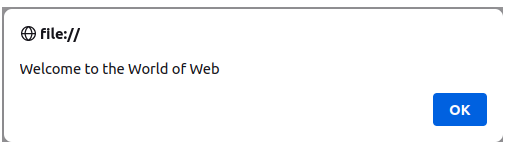
Let's examine an example of importing an external JavaScript file using the <body> tag:
index.js
scriptExample.js
Output:
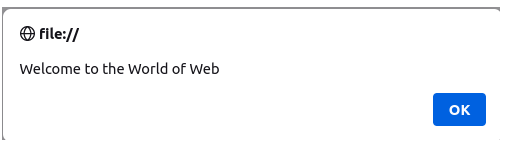
Features of JavaScript
- Lightweight: JavaScript is a small language, with a relatively small syntax. This makes it easy to learn and use, even for beginners.
- Interpreted: JavaScript is an interpreted language, which means that it is not compiled into machine code before it is executed. This makes it faster to develop with, but it can also make it slower to run.
- Object-oriented: JavaScript is an object-oriented language, which means that it uses objects to represent data and methods. This makes it a powerful language for creating complex applications.
- Dynamic typing: JavaScript is a dynamically typed language, which means that the type of a variable is not declared explicitly. This can make it easier to write code, but it can also make it more error-prone.
- Cross-platform: JavaScript is a cross-platform language, which means that it can be run on different operating systems and devices. This makes it a versatile language for creating web applications.
- Extensible: JavaScript is an extensible language, which means that it can be extended with new features and libraries. This makes it a powerful language for creating custom applications.
Applications Of JavaScript
- Creating interactive web pages: JavaScript can be used to add interactivity to web pages, such as by making buttons click or images move. This makes web pages more engaging and user-friendly.
- Building web applications: JavaScript can be used to build web applications, such as social media apps, gaming apps, or productivity apps. This makes it a powerful language for creating dynamic and interactive applications.
- Processing data: JavaScript can be used to process data from a variety of sources, such as databases, APIs, and file systems. This can be used to create dynamic content, build reports, or create analytics.
- Building real-time applications: JavaScript can be used to build real-time applications, such as chat apps, video conferencing apps, or collaborative editing apps. This allows users to interact with each other in real-time.
- Creating animations and games: JavaScript can be used to create animations and games. This makes it a popular language for creating interactive content.
- Automating tasks: JavaScript can be used to automate tasks, such as sending emails or updating spreadsheets. This can save time and effort.
- Improving the performance of web pages: JavaScript can be used to improve the performance of web pages by making them load faster and run more smoothly.
Examples of JavaScript
- Google Maps: Google Maps uses JavaScript to power its interactive maps. Users can zoom in and out, pan around, and search for locations.
- Facebook: Facebook uses JavaScript to power its user interface. Users can like posts, comment on them, and share them with their friends.
- Twitter: Twitter uses JavaScript to power its user interface. Users can tweet, retweet, and reply to other users' tweets.
- YouTube: YouTube uses JavaScript to power its user interface. Users can watch videos, search for videos, and create playlists.
- Netflix: Netflix uses JavaScript to power its user interface. Users can browse movies and TV shows, add them to their queue, and watch them.